Learning to Use Sets and Tuples
A Set is a unordered collection of items, this is mutable which means you can change the values in the set however duplication is NOT allowed. So in a set you cannot have router 1 twice.
Whereas in a Tuple it is immutable which means you can NOT change the values once it is done. However you can have duplication.
1.1 Creating and Using Sets
Int he example below, I have created a Set (now it used the curly braces{} just like dictionary, however there is no key value pairs so that is how you can tell it is a set not a dictionary). I have repeated the objects twice, but when i print the set it removes the duplicates.

1.2 Adding and Removing Elements in a Set
Using the .add() to add a new item to the Set. And also using .remove() to remove from a Set.
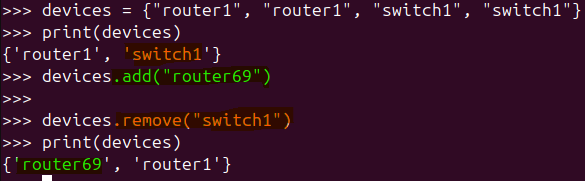
1.3 Set Operations in Network Automation
The example below is allowing you to compare the the same items in different sets. So for example you can have a set of configured devices and another set which is active. Items could be configured and active which will be in the same two sets.
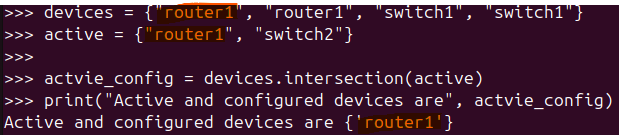
You can also differentiate with two different sets.


Another example below:


2. Understanding Tuples in Python
Rememeber Tuples are immutable which means you cannot change the values in the Tuple but you can have the same items in the same Tuple.
2.1 Creating and Using Tuples
Below example illustrates how to create a Tuple, I have included two different methods you can use to print the Tuple, using f-string and without.
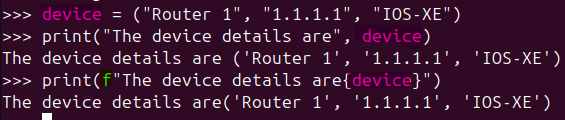
2.2 Accessing Elements in a Tuple
You can print the items/objects in the Tuple by calling the index. Remember the first object always starts with 0.
Example below, I created 2 variables and calline the tuple but with the index number.
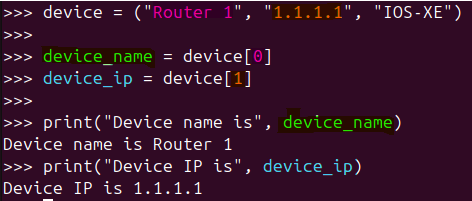
Another Example

2.3 Tuples as Keys in Dictionaries
You can add Tuples into a Dictionary, and then call the Tuples.
In the example below, I created two Tuples within a dictionary. Dictionary is highlighted in Orange and Tuples are in Yellow. I then create a variable that calle the Tuple in Green. I create new variable called ‘policy’ in Pink which will then call the dictionary and Tuple in Green, if the Tuple in the dictionary is not the same as the variable ‘pair’ in Green then print ‘No Policy defined’ in Blue.
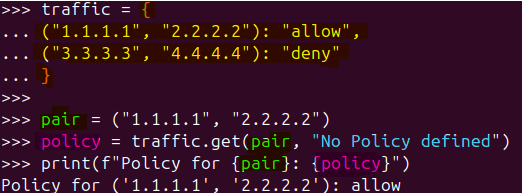
3.1 Managing VLAN Assignments with Sets and Tuples
In this example, we have existing vlans in a dictionary, we then create a Tuple with a new vlan and relevant information. A for loop basically explaining if the new vlan index 0 in Orange already exists in the existing vlan dictionary then print the vlan already is configured.
Else statement to say if it isnt in the dictionary then, to print the new vlan details specifically the indexes that is in the Tuple.
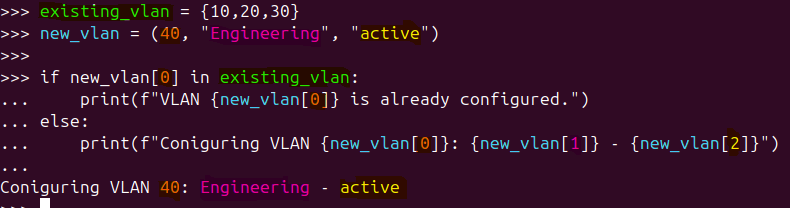
Another example:
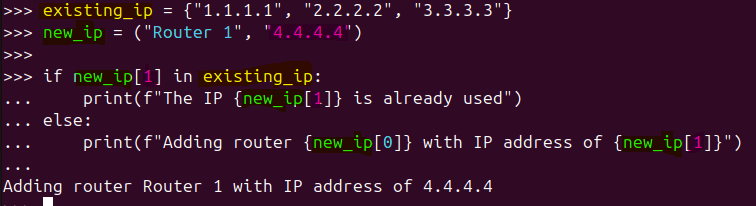