Learning to Use Strings
Section 1: Creating and Accessing Strings
To access into Python type:
python3 #current version is 3.
To exit into Python type:
exit()
or
ctrl + D #Linux/MAC or ctrl z #Windows
Creating a String:
1.2 Accessing Characters in a String
You can access a specific character of the string, this always begin at 0 as the first character:
device_name[0]
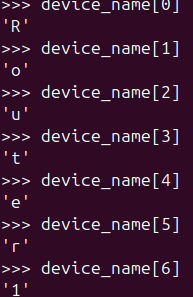
1.3 Slicing Strings
This is useful if you want to only take a section of the characters such as the first 6 characters of the String:
The example below is a String that is sliced, so I have created a String called ‘device_name’ which contains ‘Router1’. I then only want to print the characters of ‘Router’ without ‘1’. Remember string characters start from 0 which means it is ‘R’ in the example below.
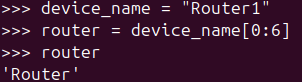
Section 2: String Concatenation and Repetition
You can create multiple Strings then add them together.
Example below illustrates how you can add two or more strings together, everytime you add a string make sure you add the + then the string.

2.2 Repeating Strings
In the example below, I have concatenated two strings together and repeated the string “no shutdown” 3 times.
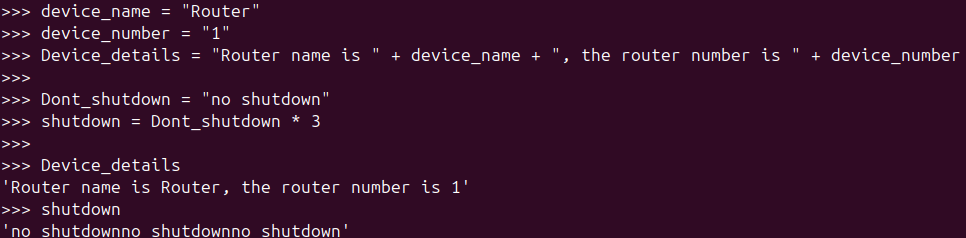
Section 3: Common Built-In String Methods
3.1 Converting Case: lower()
and upper()
This example below illustrates in how to change the String variable from Upper case to lower case and vice versa using the lower() and upper() syntax.
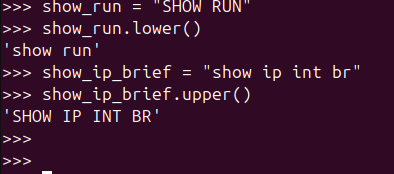
3.2 Finding Substrings: find()
You can find the specific String and see where it is positioned within the index. And by that using the example below ‘WAN’ is at the index 16 as remember index starts from 0 so ‘I’ is index 0. If you see a result of -1 this means that the string I tried to search for doesn’t exist.
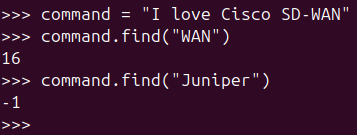
3.3 Replacing Substrings: replace()
You can replace a specific String to something else. Example below I changed the string from ‘network’ to ‘Python’.

3.4 Splitting and Joining Strings: split()
and join()
You can split the Strings up into every work per space.

You can also join the words back.

3.5 Removing Whitespace: strip()
, lstrip()
, rstrip()
You can remove empty space in the String such as leading and ending spaces of a String.
strip() = removes the leading and ending spaces together.
lstrip() = removes the leading space onlu
rstrip() = removes the ending space only.
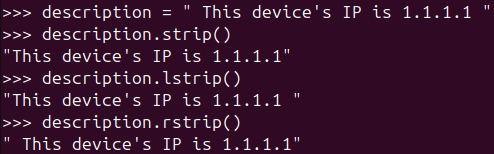
3.6 Checking String Content: startswith()
and endswith()
You can check if the specific String starts with or ends with whatever you search for in that String. The example below shows if the String begins with ‘show’ it will return the Boolean logic of true or false.
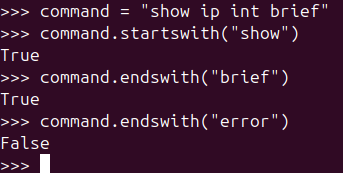
Section 4: Advanced String Operations
4.1 String Formatting
Using the keyword ‘f’ means it will format the String, so in networking you could add two Strings together by calling the indiviual String. In this example I created 2 Strings ‘ip’ and description’, i then formatted the two Strings together with a third String.
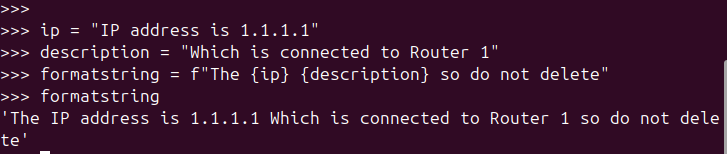
Another way to format the string is to use an alternative method. In the ‘formatstring’ String, the {} is calling for the string to format ‘.format()’ at the end of the command which is ‘ip’ and ‘description’.
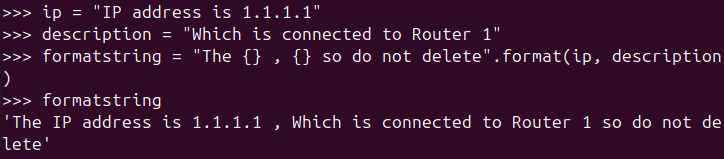
4.2 Checking for Substrings with in
and not in
You can check if a certain keyword is within the String. So if you wanted to check if the IP address is within the String you can use the Substring.
4.3 Escaping Special Characters
Using special characters that allows you to quote within a string use the \ button.

Section 5: Practical Exercises
5.1 Exercise 1: Extracting Interface Names
Write a Python snippet to extract the IP addresses.
The solution below is essentially creating three lines illustrating the IP addresses, then splitting the line but when splitting use the index 0 which belongs to ‘x.x.x.x’ IP. In my example we have three lines and each line has 3 interfaces:
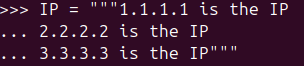
1.1.1.1
2.2.2.2
3.3.3.3
I then write the script so that it splits the lines but when splitting the lines up I want to only record the IP address and it is in index 0. Hence the script has 0, it will then call the String ‘IP’ and display into different lines(split).

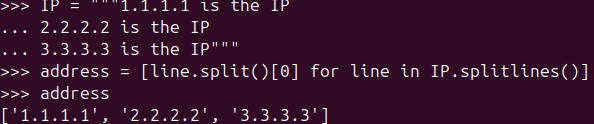
If I wanted to split the lines but record the status of interfaces whether the interface is up or down i could use this example below. Notice the index is set to 2 which means it is the last ‘word’ I am looking for. As the first ‘word’ is index 0.
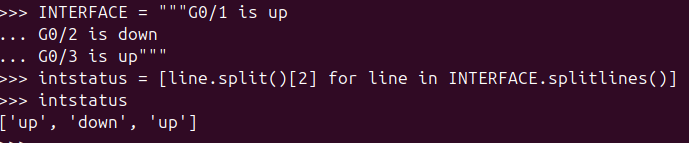
5.2 Exercise 2: Generating Configurations
Write a Python snippet to generate interface configurations for multiple interfaces using a loop.
I create the list of interfaces first (remember list starts with square brackets and ends in square brackets [].

I then create a loop which calles the List ‘interfaces’, the loop is called ‘THEINTERFACE’. When the loop is called two new lines is being added with the String for the description and not to shutdown the port. Lastly the loop asks the script to print out the config.

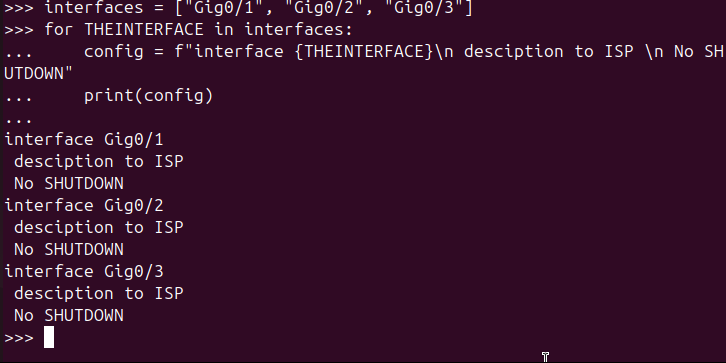
5.3 Exercise 3: Parsing Command Output
Write a Python snippet to parse the output of the show ip interface brief
command and extract interfaces with the status “up”.
First off i create the List and called it ‘showipint’.
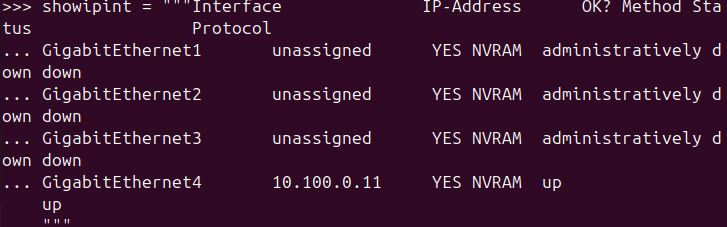
I then split the lines but this time I split the lines that begin with my interface (GigabitEthernet0/X) and IF it includes ‘up’ within the same LINE display it.

Alternatively, you could do the same for if the Status is Administratively down then display the interface.
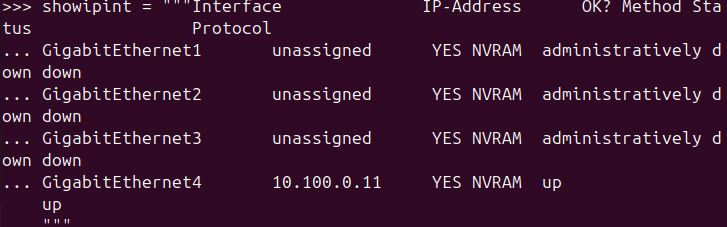