Learning to use While and For Loops
1. The for
Loop
‘for’ loop is used to go through the sequence such as a list, dictionary, tuple, string or range which then exectures the code until the loop finishes. Such as a list of devices and configs.
1.1 Basic for
Loop
The ‘device’ word is basically creating a holding place which is where the ‘for’ loop is called/created, then the loop called ‘device’ will then call the ‘list’ names ‘devices’ and in that list contains the list of devices. It will then print the loop called ‘device’ and that contains the list of ‘devices’ (Router 1 etc). ‘device’ could be anything as you are just naming the ‘for’ loop.
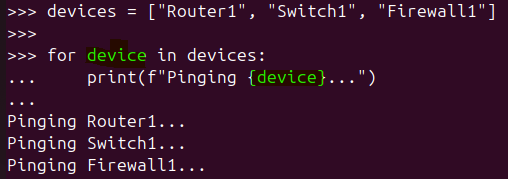
I have another example which I have renamed the ‘for’ loop to be TEST as an example where it can be anything, but once you have named the loop, and you want to display anything related to the loop it must match. It is always recommended to have a good naming system so it makes sense.
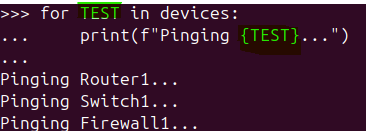
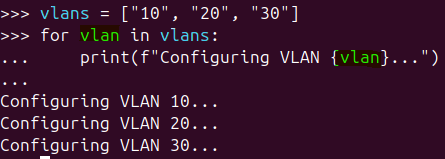
1.2 Using range()
with for
Loops
using ‘range()’ keyword allow you to repeat the loop over again with x amount of times.
In the example below, I created a ‘range(10) which means repeat this process 10 times, however you will see in the f-string I called the loop ‘pinging’ with a + 1. the reason is remember the indexing where it will always start with a 0 not 1. If I didn’t add the ‘+ 1’ it will begin with “Pinging 0 for Router 1”. So to make this more realistic as an example we started with ‘+ 1’
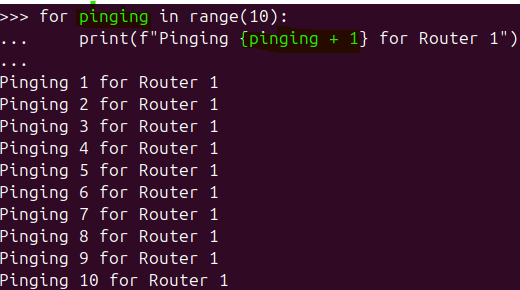
1.3 Nested for
Loops
This is used when you create a ‘for’ loop and to call another loop within the loop. so this runs a loop inside a loop.
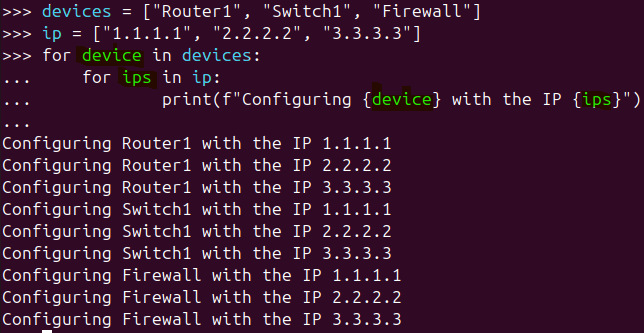
2. The while
Loop
You use the ‘while’ loop based on a specific condition, which will be repeated until it is no longer ‘true’. A good example would be keep ping the device while it is offline, until it becomes online then stop the ping.
2.1 Basic while
Loop
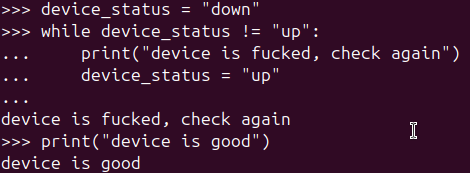
2.2 Infinite Loops and Break Statement
‘break’ keyword can help stop a while loop prematurely.
The example below, sets the ‘attempt’ to 1, so the first iteration it already sets it to 1 which will then display “Pinging attempt 1”, the loop then incremements 1 so ‘attempt’ is now 2, it will run through the 2nd iteration and displays “Pinging attempt 2”, it will add another 1 to it so it makes it 3, the third iteration will repeat itself and display “Pinging attempt 3”. an increments 1 which ‘attempt’ is now 4, now the loop becomes ‘True’ (Boolean) which the nested ‘if’ statement comes in if it is more than/greater than 3 then display “Maximum attempts reached. stopping”. The ‘break’ keyword stops the loop.
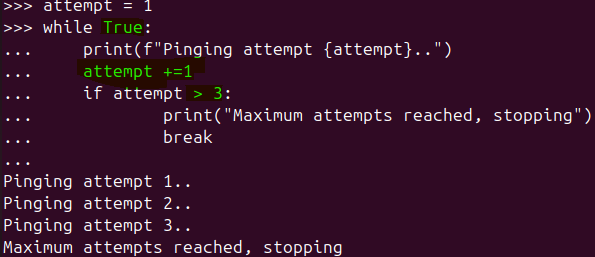
3. Controlling Loops with break
and continue
‘break’ as descrived previously breaks the/stops the loop but with ‘continue ‘ you can carry on with the loop and moves onto the next iteration.
3.1 Using break
in Loops
The example below, we create a list which has the status of down and up.
We then created a for loop called ‘status’ and call in th elist created ‘device_status’. Nested ‘if’ statement checks through different iterations until there is a match. Because the first and second iteration it is ‘down’ based on the list (device_status) it will continue to run through the loop until a match is found if it is ‘up’. Once it is ‘up’ then the ‘break’ comes into play.
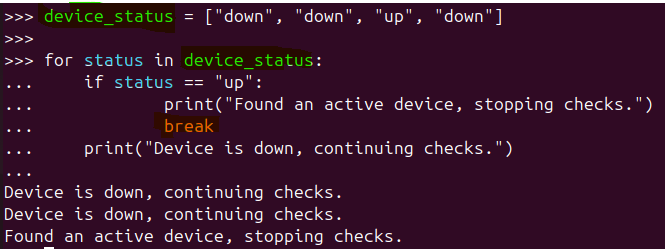
3.2 Using continue
in Loops
‘continue’ statement will skip the rest of the loop onto the next iteration.
In this example below, it will look for the status that matches up from the list. Once it has a match it will skip to the next iteration until there ISN’T a match for (“up”). So with the first iteration, the loop doesn’t have a match for “up” so it prints the message, the 2nd iteration matches “up” so it will skip this iteration until it goes through all the items in the list.
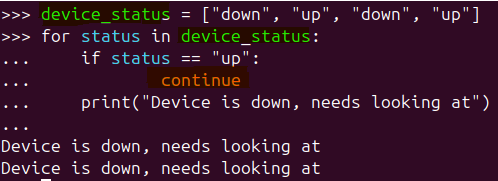
4. Nested Loops in Network Automation
You can use nested loops for more complex operations and using different parameters to different devices.
4.1 Basic Nested for
Loops
The example below is a nested for loop in a for loop. It will loop the ‘devices’ list then the 2nd list called ‘configs’. So it will start with ‘Router1’ and ‘IP’, ‘VLAN’ and ‘Hostname’ then ‘Switch1’ and so on.
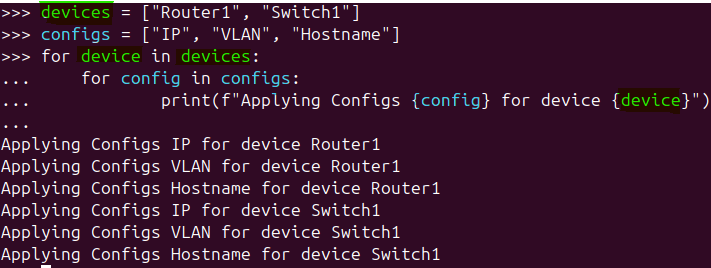
5. Practical Use Cases of Loops in Network Automation
Using Loops is very helpful when configuring a number of devices.
5.1 Automating Device Configuration
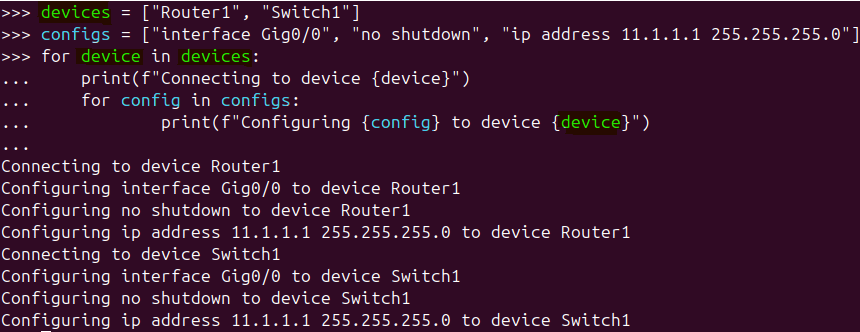