Learning to Use Dictionaries
Dictionaries are used to store in Key Value pairs (I briefly touch up on this subject in the using if, elif and else statement section). Useful in managing configs, storing device info.
1. Creating and Accessing Dictionaries
You can use curly braces {} or brackets () when creating dictionaries.
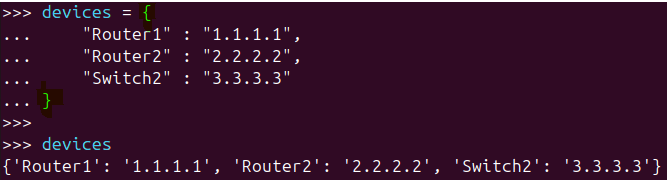
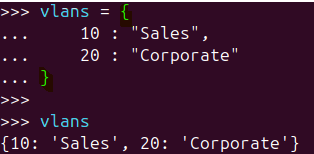
1.2 Accessing Dictionary Values
You can store the value from the key by stating which key so it stores teh value.
I created the dictionary on the example above calle ‘devices’ which I then wanted to store the IP address of ‘Router1’.
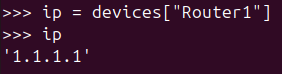
Anothe rexample is accessing the VLAN number which will then tell me what VLAN it belongs to.
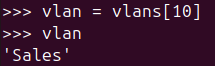
The below example illustrates in calling out the Key from the dictionary and then printing the value. In the example below I will store the key pair for ‘Router’ 3 and then printing out the IP address of it.
I first create the dictionary below:
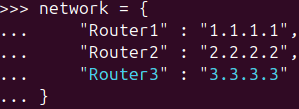
I then store the Key pair first. and calling it ‘ip’

I then display the message and call the value pair by using f-string as one method below:

Another method is to call the variable directly ‘ip’.

2. Modifying Dictionaries
You can modify or add key-value pairs.
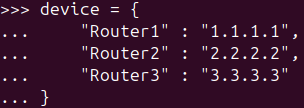
I will now add a new key-value pair to the existing dictionary ‘device’.

I can also modify the value pair and changing the ip from ‘4.4.4.4’ to ‘9.9.9.9.’

2.2 Removing Key-Value Pairs
I create a couple of dictionaries first to use as examples:
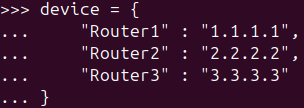
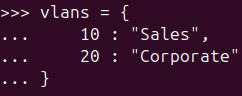
Using the same dictionary called ‘device’ from above, I deleted ‘Router2’ from the dictionary.


I also can use the pop() method. I create a new variable called ‘del_vlan’ and then removed VLAN ’20’ from the ‘vlans’ dictionary.

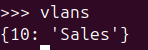
Below example
- In pink is removing the key ‘Router1’ and value ‘ 1.1.1.1’ pair from an existing dictionary called ‘device’
- In Blue, it is adding another key value pair into the existing dictionary.
- In Orange, it is editing the value from ‘3.3.3.3’ to ‘33.33.33.33’.

3. Iterating Through Dictionaries
Using the dictionary example for this section.
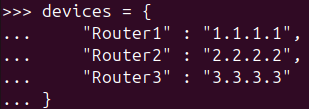
3.1 Iterating Through Keys
You can go through the dictionary in iteration, so one after another using ‘for’ loops. Example below will call the key from the dictionary. And the dictionary key is router names.
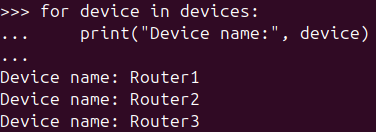
3.2 Iterating Through Values
Now instead of just calling out the keys in the dictonary, you can just print out the value instead which is the IP addresses.
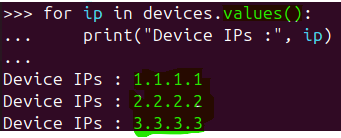
3.3 Iterating Through Key-Value Pairs
You can call out the key value pairs at the same time using .items().
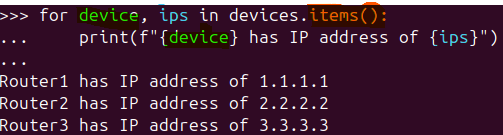
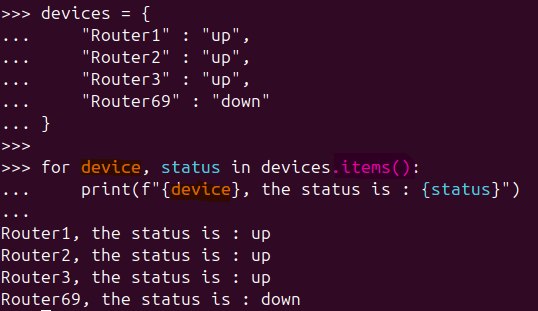
4. Nested Dictionaries
4.1 Creating Nested Dictionaries
You can include more than one key pair by using nesting.
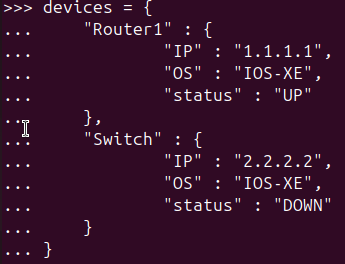
4.2 Accessing Nested Dictionary Values
The below example will store the ip address of the router ‘1.1.1.1’ and when you print it you can see the result.

Another example to find the OS version of the switch you can use a similar example:


5. Dictionary Methods
5.1 get()
Method
The get method is used to retreive specific key. The example below is looking for the ‘Router2’ and if it is not there then print ‘not available’.

5.2 keys()
and values()
Methods
To print all the keys in a dictionary example below, will list your keys from the dictionary. ‘list’ highlighted in blue is a keyword.

And to obtain the values from a dictionary:

Below is another example where if you use the get method and the item in the dictionary is not available then you can print an alternative message. Router 69 is not in the dictionary therefore it will print ‘Not Available’.
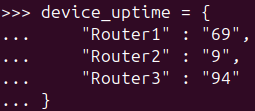

6. Combining Dictionaries
6.1 Using update()
Method
You can add another dictionary you created into an existing dictionary using the update() method. Example below I have created a ‘devices’ dictionary and added a new dictionary together called ‘devices2’. So now ‘devices’ dictionary in Blue will include the key value pairs from ‘devices2’ in Green.
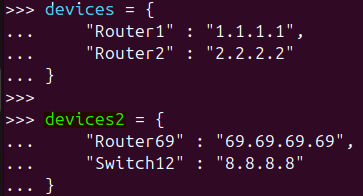


6.2 Merging Dictionaries with **
Operator
You can merge two dictionaries together:

However, ‘devices’ and ‘devices2’ will still hold the existing key value pairs. You would need to call the ‘merge_dict’ if you wanted the two dictionaries.